Implementing React hooks/Redux with axios the Right Way Part 2

Update: This is the second part in a two-part series. You should read the first part first.
In Part 1, we simplified the Implementaion of the Redux Boilerplate by breaking down each components involved and showing how each work together, we used Axios to make Http Request along side react hooks like useDispatch, useSelector and useEffect. We used fake data from fakestoreapi to fetch list of products and SemanticUI for styling.. (If you haven’t already read part 1, read it now! to get up to speed).
This time, we are going to do something really cool — when we click on a product we would be getting the product details in another page and display that in our project. Yes!!! 😉 you guessed it right👍, we will be achieving that with the help of the react-router-dom to link the pages.
Just Like in Part 1, an addition to the requirement will be the react-router-dom.
Requirements
- Now open up the app we created in Part 1 as we are going to continue from where we stopped and start the app.
npm start
2. Your app should look like this when finished building.

3. If you recall in Part 1, we already declared two action Types
SELECTED_PRODUCT which monitors products selected and
REMOVE_SELECTED_PRODUCT which removes the previously selected product from store when new product has been selected.
we imported them in our productReducer.js and used them in the selectedProductReducer function.
in the SELECTED_PRODUCT case we are returning whatever state we have declared using the spread operator (…state) after that we destructure the payload (...payload) and set the default to the already existing state.
In just a minute I'll come back to why we have the REMOVE_SELECTED_PRODUCT case.
4. Also in Part 1 we created the selectedProduct and removeSelected function in the productActions.js to help with with the dispatch of our actions.
5. If you haven’t created the Products details page yet, right click on the containers folder and create a file with the name ProductDetail.js

6. Now we need to install the dependencies for the react router by running the command below.
npm install react-router-dom
We will be Linking the Products Page to the Product Details page using the React Router
7. Next Open up your ProductComponent.js. We will be importing a Link tag from the react-router-dom.
Notice the {`/product/${id}`} above, we are passing the {id} as parameter to the Link tag so as to route us directly to the specific product selected so we can then fetch that particular product by id in the ProductDetail Component.
8. Having added a link to the ProductComponent page, Open up your ProductDetail.js Component and paste the code below.
From the Code above, we imported the useParams hook from react-router-dom, we also imported axios to help with the API call, useDispatch and useSelector redux hooks and lastly, the useEffect react hook.
We are able to extract the parameter value sent from the ProductComponent when we clicked on a Product using the;
const {productId} = useParams();
Now that we are able to extract the parameter value we can now pass it to the API call we’ll be making with axios to fetch details for that particular product based on its ID.
Next we made use of the useDispatch hook for dispatching the selectedProduct action created in the productActions.js in No 4. above.
After that, we imported the useSelector hook to help us access the selectedProduct. Recall that we created the selectedProductReducer in the ProductReducer.js above. Hence, the useSelector in the ProductDetail is going to get us the state and then return the product in the state.
Next, we use the useEffect hook to call our function fetchProductDetail.
Note: The conditional statement there.
if(productId && productId !=="") fetchProductDetail();
To avoid any error, the useEffect will only run if we get the productId from the useParams hook and also if the productId is not an empty value.
Lastly, we destructure the data gotten from the product state so as to display it in the UI
Notice that; in the return statement we have;
{Object.keys(product).length === 0 ? (<div>...Loading</div>) : (...)}
We are first showing the …Loading text when the product length == 0, that is, when the product is empty (at that point we are trying to fetch the data of that product from the API). So once the product has been fetched we then continue to display the product details.
9. in №4 above i mentioned that we were going to look at why we have the REMOVE_SELECTED_PRODUCT action type in the selectedProductReducer function in the productReducer.js.
case ActionTypes.REMOVE_SELECTED_PRODUCT:return { };
What we have in the selectedProductReducer is that we are first fetching the previous state and then populating it with the new state (No 2 above). Hence, the previous product selected will still be displaying in the UI before the new product gets fetched which is invalid.

Now, that is to help us clear out the selected product from the state when the ProductDetail Component gets destroyed (i.e when we route out of it).

so that whenever we call the removeSelectedProduct action we will return an empty state.
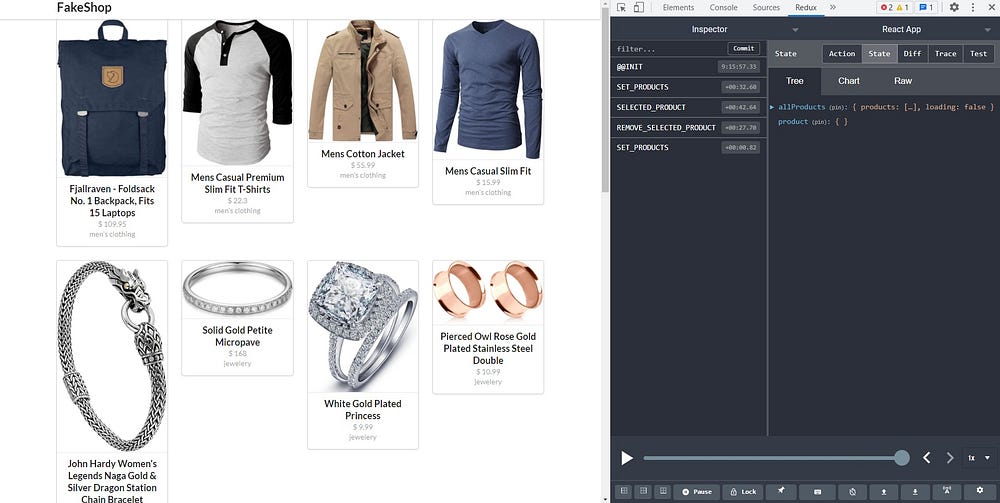
Therefore we added the action to the useEffect hook in the ProductDetail Component in No 8 above.
if(productId && productId !=="") fetchProductDetail();return () => {dispatch(removeSelectedProduct())}
and that's all. We now have a fully working React Application with redux for state management.
Here is the Link to the code on GitHub https://github.com/MindInitiatives/FakeShop
Don’t forget to Follow me to see more of stories. Thanks😁😊 and Enjoy !!!