Implementing React hooks/Redux with axios the Right Way Part 1

Hey Guys, So in this Tutorial we will be learning how to use react hooks with redux the right way. There are so many tutorials out there about how to implement this but most of them just end up making it look complicated, Therefore, We will be Implementing the Redux Boilerplate in the simplest and understandable way, Also we will be using Axios to make Http Request along side some react hooks like useDispatch, useSelector and useEffect. We will use fake data from fakestoreapi to fetch list of products and when we click on a product we would also be getting the product details (Part2) and display that in our project. Enough Talk, Lets delve right into it.
Requirements
- Firstly, I will assume you already have a react app created. If not, from your terminal type in the command below. I am going to name my app “react-redux-tut” you can name yours whatever you want to.
npx create-react-app react-redux-tut
2. Install all the required dependencies by running the command below.
npm install redux react-redux axios
3. Now go to your src directory and create two directories with the names containers and redux. I know what you’re thing now😉 . and you’re right!👍. Our Components are going to be in the containers directory while everything that has to do with redux will be in the redux directory.
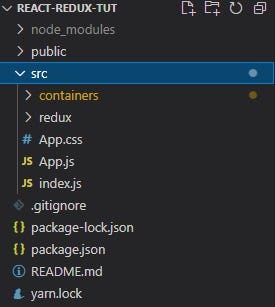
4. Now go to your redux directory and create store.js. also, create three more directories with the names actions, reducers and constants and inside the constants directory create action-types.js file.
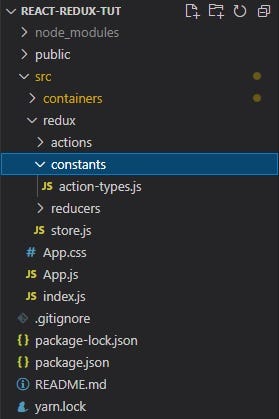
5. Now we declare some types in our action-ypes.js file. So open the file and paste the below code.
SET_PRODUCTS is for getting the list of products,
SELECTED_PRODUCT monitors products selected,
REMOVE_SELECTED_PRODUCT is to remove the previously selected product from store when new product has been selected. we will discuss more on this in the later part of this article,
PRODUCTS_ERROR tells us if an error has occurred while making request to our API.
6. Now it’s time to configure our store. So open your store.js file and paste in the code below. Notice that we have window.__REDUX_DEVTOOLS_EXTENSION__ && window.__REDUX_DEVTOOLS_EXTENSION__() it is for us to be able to monitor our store from the browser. you can checkout this link for more details.
We are first importing the createStore method to create our store. We have also imported index from our reducer directory which we will create in the 8th step.
7. Next, For us to be able to see and monitor our redux store in the browser we need to install the extension from the chrome web store.

Once Successfully installed we should see something like this in our list of extensions when we type in “redux” into the search bar.

8. Now go to your reducers directory and create a file with the name index.js and paste the below code.
This file contains all our reducers in one place. We are first importing our combineReducers method from redux so that we can merge all our reducers which we are importing. we are also importing productReducer and selectedProductReducer which we create in the next step.
9. Inside your reducer folder create a file with the name productReducer.js and paste the below code.
So here we are first importing our ActionTypes which contains our constants SET_PRODUCTS and SELECTED_PRODUCT from our action-types.js file. After that, we are initializing some default states for our products and then we are creating a function and inside that, we are using a javascript switch case. The SET_PRODUCTS case is first returning an object of all the previous state we have declared using the spread operator (…state) after that the products object contains our actions data (action.payload). Now if suppose there is no response from the API or there is an error in calling the API the PRODUCTS_ERROR case will set the loading to false and the error will display the error message we will send from our action file. By default, we are calling the state which contains our initialState. Now we will create our action file in the next step but before moving into the next step we will see that whether our chrome devtool is working or not so open your root index.js file and import Provider from react-redux and store from store.js file and then wrap your App component with Provider and pass store as props to store.
Now start your app npm start
, open your chrome developer tools ctrl + shift + c
and go to the redux tab and click on state to see the initial state we declared in our productReducer.js file.

10. Now open your actions folder and create a file with the name productActions.js and paste the below code.
This file is responsible for creating all our actions like here it’s creating an action that will help us to fetch data from the API using the Axios library which we’ll be showing next. So here we are importing all our ActionTypes constants from the action-types.js file.
Note: the payload contains the data which will be passed to the productReducer which is then getting imported to the index.js file which contains products object inside combineReducer method. So ultimately products object inside our combineReducers method contains all our data.
Our redux boilerplate is set up and now it’s time to actually use our data.
11. Now that it’s time to start creating our components. we need to add SemanticUI for styling. Go to your public directory and open your index.html file. in the link section, paste in the SemanticUI CDN
<link rel=”stylesheet” href=”https://cdnjs.cloudflare.com/ajax/libs/semantic-ui/2.4.1/semantic.min.css" integrity=”sha512–8bHTC73gkZ7rZ7vpqUQThUDhqcNFyYi2xgDgPDHc+GXVGHXq+xPjynxIopALmOPqzo9JZj0k6OqqewdGO3EsrQ==” crossorigin=”anonymous” referrerpolicy=”no-referrer” />
12. Remember that in Step 3 we created a directory with the name containers go to that directory and create Header.js (which will hold our header in the app), ProductListing.js (which will contain the logic for fetching the products we have in our redux store which we will be fetching from the API.), the ProductComponent.js (which is the initial component that holds the product item) and the ProductDetails.js (which we will be working on in the Part2 of this story)

Now Open Header.js and paste in the code below. (Note: The classes are all SemanticUI classes. Click on this link to see more about SemanticUI
13. Next, Open ProductListing.js and paste in the code below
So here we are importing useDispatch and useSlector hooks from react-redux and our setProducts function from our productActions file. Now inside our functional component, we are passing our useDispatch hook to the dispatch variable.
After that, we are creating an asynchronous function fetchProducts which is passing dispatch as a parameter and then using Javascript try catch for catching the response from API. If API sends the response without an error it’ll be caught in try and data will be passed to the payload and if there is any error it’ll be caught in catch and response will be passed to the payload.
This dispatch is then called inside our react useEffect hook in the first parameter with setProducts as an argument. This will render our fetchProducts function to fetch data from the API. Remember that setProducts takes an argument of products from our productActions.js which is supposed to be the payload gotten from the API response. After that, we are using the useSelector hook to extract data from the store. So we are passing our state to the products variable. Now if we console our products variable we will find all our states like loading, and products.
Then, for us to be able to use this data in our ProductComponent.js we need put it in a return statement from ProductListing.js
Note: state inside useSelector is declared in our reducer index.js file. This holds all our products state. If we console.log the state inside useSelector: useSelector(state => console.log(state)) you will get all the states declared in our combineReducer. So this way you can get data from the store to any of your component just call the action file function to that component and use useSelector to call the state to that component.
14. Next, we need to get the data exported from ProductListing.js so we can use it in out ProductComponent.js. Open ProductComponent.js and paste in the code below.
Here, we are using the useSelector hook to extract data from the store. We are then destructuring our product and iterating our products array with the javascript map function. To use our product item we are passing it as a variable renderList so we can add it in the return statement to display in our App.js
15. That’s it, now import your products component to App.js and refresh the browser to see changes.

PS: In the Part2 of this App I'll be creating a details page opens a page with the details of a product whenever you click on the product. Also I will be dropping the link to the repo.
Don’t forget to Follow me to see more of stories. Thanks😁😊 and Enjoy !!!